Using Firebase Database (Web)
The Firebase database is a NoSQL, real-time, and cloud-hosted database.
Data is stored as JSON and synchronized in real-time to every connected client.
The following steps show how to connect a web page to a Firebase database:
- Visit the Firebase Console page to configure your project, and then click the “Add project” hyperlink.
- Give a name to your project, and then click the “Continue” button.
⇓
- Choose “Default Account for Firebase,” and then click the “Create project” button.
- Your project is created and you are now good to go.
- Click the 3rd icon (</>) that’s for the Web.
- Give a nickname to your web app, and then click the “Register app” button.
- You will see the configuration of your app like below.
Note that this code is incomplete since it lacks the command such as:
databaseURL: "https://fir-6-a29e0-default-rtdb.firebaseio.com",
|
It may be because the storage is not created.
Click the “Continue to console” button.
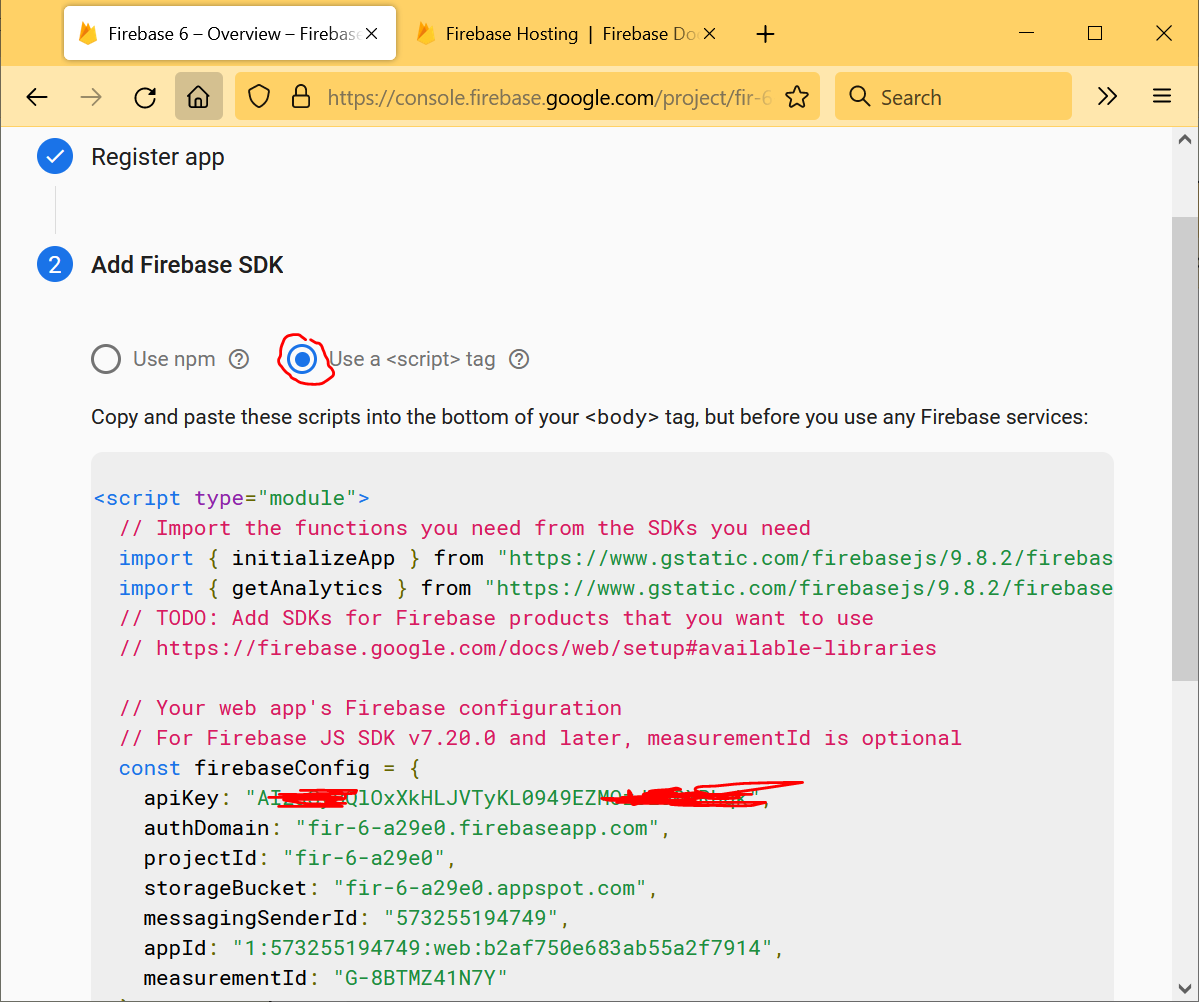
- Select the “Realtime Database” item on the left pane, and then click the “Create Database” button.
- Select the “test mode.”
Otherwise, your code has to include authentication.
Click the “Enable” button.
- Activate the Firebase Storage by selecting “Storage” item on the left pane and clicking the “Get started,” “Next,” and “Done” buttons.
- Go back to the Firebase Console, and click the symbol
</>
:
At the web app nickname such as 525-1, check Project Settings:
Now the databaseURL
value is available.
Do NOT use the following script if you are not using Firebase Analytics.
Otherwise, it will mess up your code, which makes your code invalid even if you undo the changes, and you have to recreate the code.
Copy the code below and add the following line after the constant firebaseConfig
definition:
firebase.initializeApp( firebaseConfig );
|
Create an HTML page and paste the configuration code which you copied in the previous step.
ⓐ: Do not know why copying and pasting the code below does not work.
Instead, use the source code from the
demo page.
ⓑ: Note that the web app works, but it only works for 30 days by picking the Test Mode.
|
http://undcemcs01.und.edu/~wen.chen.hu/library/firebase-web/demo.html
|
|
<!DOCTYPE html>
<html lang="en">
<head>
<script src=
"https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js">
</script>
<script src= "https://www.gstatic.com/firebasejs/3.7.4/firebase.js">
</script>
<title>Managing Firebase data</title>
</head>
<body>
<center><h3>Managing Firebase Data</h3></center>
<form id="contact">
ID: <input type="text" size="3" id="ID" value="1" />
Name: <input type="text" size="30" id="name" value="Poke Mon" />
Email: <input type="email" size="30" id="email" value="poke@example.com" />
<p id="result">[result]</p>
<button type="submit" onClick="addUser( ); return false;">
Add a user</button> (required: ID, name, email)
<button type="submit" onClick="showUser( ); return false;">
Show a user</button> (required: ID)
<button type="submit" onClick="showAll( ); return false;">
Show all users</button>
<button type="submit" onClick="deleteUser( ); return false;">
Delete a user</button> (required: ID)
<button type="submit" onClick="deleteDB( ); return false;">
Delete the database</button>
<button type="submit" onClick="updateUser( ); return false;">
Update a user</button> (required: ID)
(May need to click on the Update button a couple of times to make it work. 😵)
<button type="reset">Reset</button>
</form>
<script>
// For Firebase JS SDK v7.20.0 and later, measurementId is optional
const firebaseConfig = {
apiKey: "AIzaSyDxv7m8s1SSWamfWnNVt0TXe9WO75QvDQI",
authDomain: "fir-8-dd4ef.firebaseapp.com",
databaseURL: "https://fir-8-dd4ef-default-rtdb.firebaseio.com",
projectId: "fir-8-dd4ef",
storageBucket: "fir-8-dd4ef.appspot.com",
messagingSenderId: "6825335070",
appId: "1:6825335070:web:5b4da332f1e2fc423d510f",
measurementId: "G-0E1QNR5M6R"
};
// Initialize Firebase.
firebase.initializeApp( firebaseConfig );
var usersRef = firebase.database( ).ref( 'users/' );
//
// Add a user.
//
function addUser( ) {
// Get the input values.
var ID = getInputVal( 'ID' );
var name = getInputVal( 'name' );
var email = getInputVal( 'email' );
firebase.database( ).ref( 'users/' + ID ).set( {
name: name,
email: email
} );
var out = "Added: " + ID + ": " + name + ", " + email;
document.getElementById( 'result' ).innerHTML = out;
return( false );
}
//
// Function to get form values
//
function getInputVal( input ) {
return document.getElementById( input ).value;
}
//
// Show a user.
//
function showUser( ) {
var ID = getInputVal( 'ID' );
var ref = firebase.database( ).ref( 'users/' + ID );
ref.on( "value",
function( snapshot ) {
const data = snapshot.val( );
var out = "ID: " + ID;
out += "Name: " + Object.values(data)[1];
out += "Email: " + Object.values(data)[0];
document.getElementById( 'result' ).innerHTML = out;
},
function ( error ) {
document.getElementById( 'result' ).innerHTML = "Error"; }
);
return( false );
}
//
// Show all users.
//
function showAll( ) {
var ref = firebase.database( ).ref( 'users/' );
ref.on( "value", snapshot => {
var out = "";
snapshot.forEach( child => {
out += "ID: " + child.key;
var ref1 = firebase.database( ).ref( 'users/' + child.key );
ref1.on( "value", snapshot => {
snapshot.forEach( child => {
out += child.key + ": " + child.val( );
} )
} )
} )
document.getElementById( 'result' ).innerHTML = out;
} )
return( false );
}
//
// Delete a user (delete is a reserved word).
//
function deleteUser( ) {
var ID = getInputVal( 'ID' );
var userRef = firebase.database( ).ref( 'users/' + ID );
userRef.remove( );
var out = "User " + ID + " deleted";
document.getElementById( 'result' ).innerHTML = out;
return( false );
}
//
// Delete the database users (delete is a reserved word).
//
function deleteDB( ) {
var userRef = firebase.database( ).ref( 'users/' );
userRef.remove( );
var out = "Database users deleted";
document.getElementById( 'result' ).innerHTML = out;
return( false );
}
//
// Update a user.
//
function updateUser( ) {
// Get the input values.
var ID = getInputVal( 'ID' );
var name2 = getInputVal( 'name' );
var email2 = getInputVal( 'email' );
var name3 = "";
var email3 = "";
var ref = firebase.database( ).ref( 'users/' + ID );
ref.on( "value",
function( snapshot ) {
const data = snapshot.val( );
name3 = Object.values(data)[1];
email3 = Object.values(data)[0];
if ( name2 ) { name3 = name2; }
if ( email2 ) { email3 = email2; }
ref.set( { name: name3, email: email3 } );
var out = ID + ": " + name3 + ", " + email3;
document.getElementById( 'result' ).innerHTML = out;
},
function ( error ) {
document.getElementById( 'result' ).innerHTML = "Error"; }
)
return( false );
}
</script>
</body>
</html>
|
Start testing the web app.
⇓ (after adding Poke Mon from the Web)
⇓ (after adding Digi Mon from the Web)
⇓ (after adding Sponge Bob from the Web)
⇓ (after showing the User ID #2 from the Web)
⇓ (after showing all users from the Web)
⇓ (after deleting User ID #2 from the Web)
⇓ (Updating Sponge Bob’s email to sponge.bob@und.edu from the Web)
⇓ (after deleting the database from the Web)
Q: What’s the hottest letter in the alphabet?
A: ‘B’, because it makes oil…Boil!
|